You can use the modulo operator to wrap around items. This will loop back in both directions.
# Previous item
current_index = (current_index + items_length - 1) % items_length
# Next item
current_index = (current_index + 1) % items_length
The most popular use would be handling navigation in image viewers.
Here is a simple visualization.
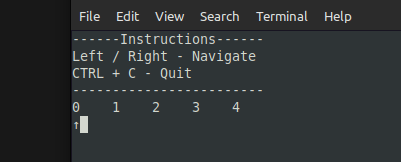
Below is the full code for this demo. It uses only the stock Python libraries.
#!/usr/bin/python3
import curses
from curses import wrapper
def main(stdscr):
images_len = 5
cur_index = 0
instructions = [
"------Instructions------",
"Left / Right - Navigate",
"CTRL + C - Quit",
"------------------------",
]
for i, instruction in enumerate(instructions):
stdscr.addstr(i, 0, instruction)
for i in range(images_len):
stdscr.addstr(len(instructions), i * 5, str(i))
stdscr.addstr(len(instructions) + 1, cur_index * 5, f"\u2191")
try:
while True:
char = stdscr.getch()
stdscr.move(len(instructions) + 1, 0)
stdscr.clrtoeol()
if char == ord('q'):
break
# Loop through items
match char:
case curses.KEY_LEFT:
cur_index = (cur_index + images_len - 1) % images_len
case curses.KEY_RIGHT:
cur_index = (cur_index + 1) % images_len
stdscr.addstr(len(instructions) + 1, cur_index * 5, f"\u2191")
except KeyboardInterrupt:
return
if __name__ == "__main__":
wrapper(main)